Crop and Upload Image Service
Image service
Simple tool to upload and edit pictures. The front-end is using in NextJs and Backend NodeJs.
NodeJs is used to be able to easily test and iterate on localhost. Production level solution will be released fully on NextJs with S3 as storage, later on**.**

https://github.com/4rokis/image-service-example
Upload new Image
Client
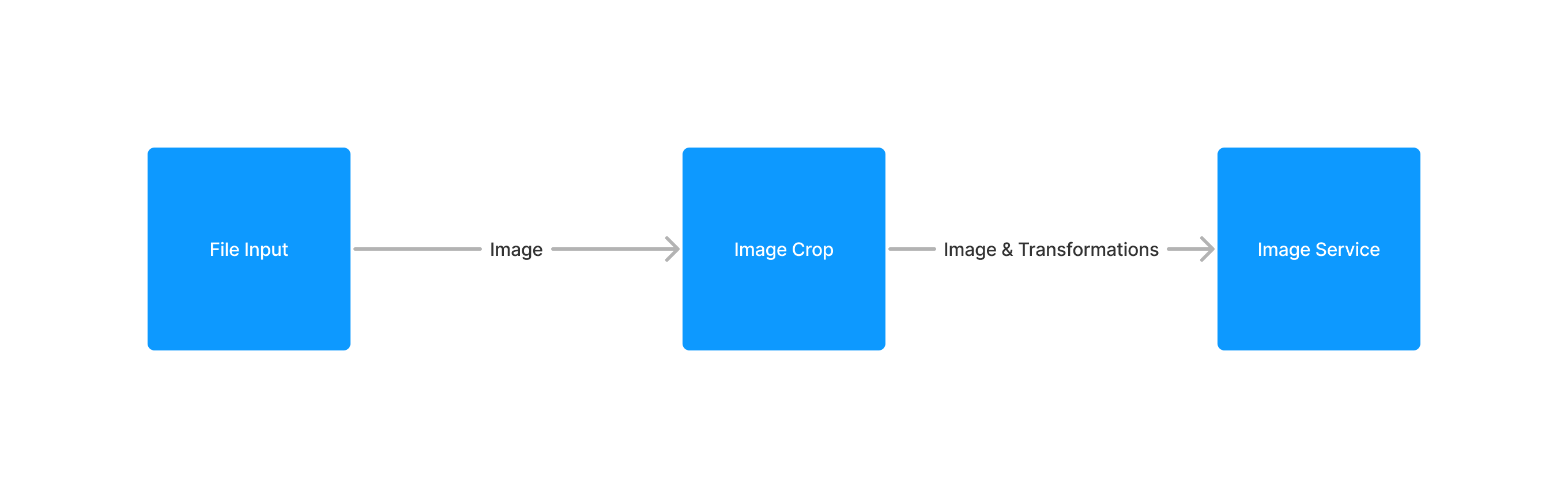
Once a user selects an image, he can modify the image(crop, zoom, rotate) via react-easy-crop library.
Once finished the original file and transformations data are passed to the ImageService.
Backend
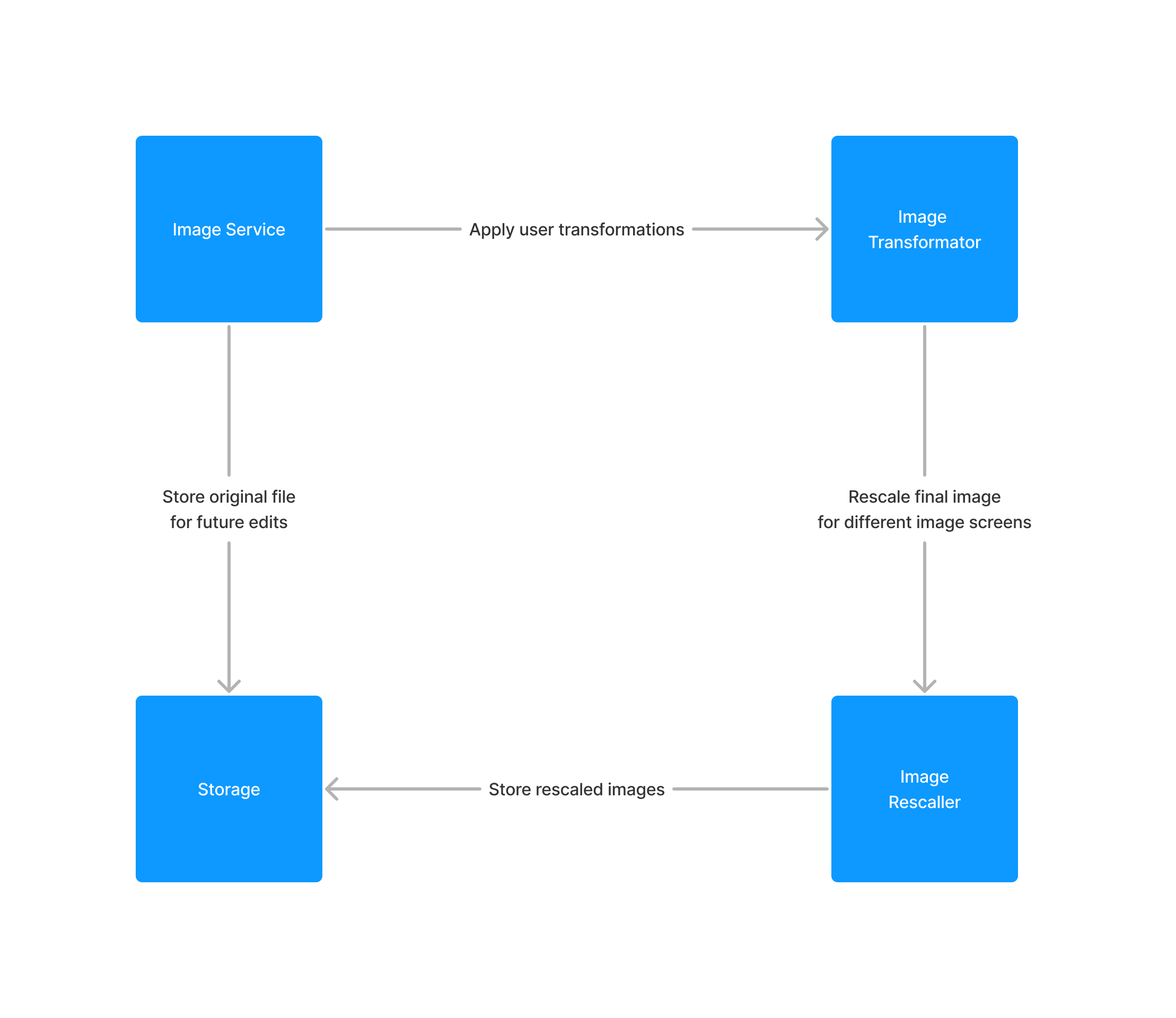
Image service accepts an image and saves it to storage (Local files, S3, Firebase, …) and takes the image, and applies used modifications (crop, zoom, rotate). The modified image is then saved in multiple sizes/scales (160, 320, 640, 750, 828, …) for a user to fetch the desired size based on their screen size.
Fetch uploaded Image
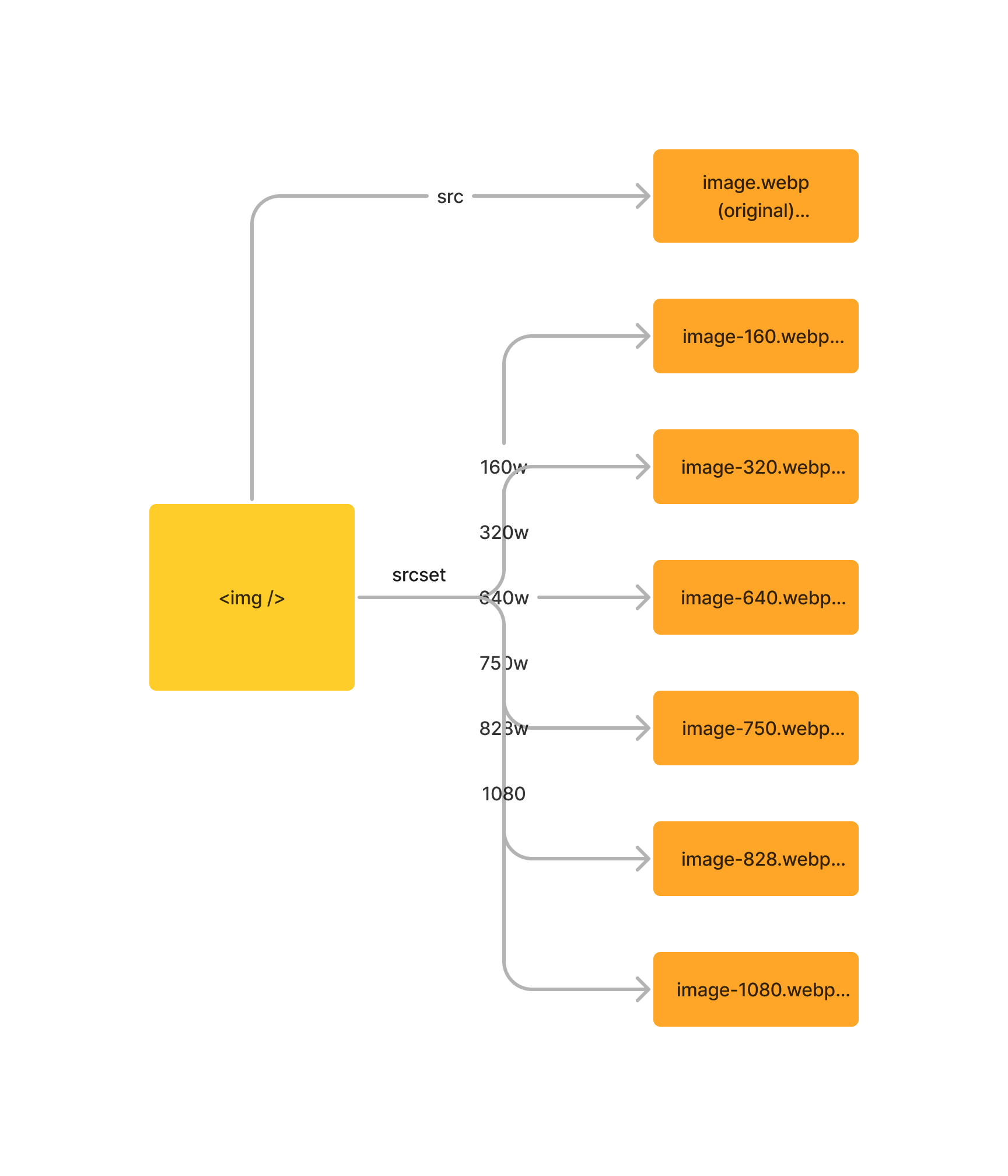
ImageService returns the image path on success. Client then can use NextImage with a custom loader
import NextImage from 'next/image'
const parseFileName = (path) => {
const pathParts = path.split('/')
const filename = pathParts[pathParts.length - 1]
return filename.split('.')
}
const myLoader = ({ src, width }) => {
if (!src) {
return ''
}
const [path, params, name, end] = parseFileName(src)
return `${IMAGE_BASE_URL}/${name}-${width}.${end}`
}
export const Image = ({ className, src, sizes }) => {
return <NextImage {...rest} loader={myLoader} src={src} sizes={sizes} />
}
or native image with srcSet
import React, { HTMLAttributes } from 'react'
import { getSrcSet } from './utils'
export const Image = ({ src, sizes }) => {
return <img src={src} sizes={sizes} srcSet={getSrcSet(src)} />
}
Image.displayName = 'Image'
to fetch the image.
The page then can use sizes prop to define what is the actual image size.
<Image src={image} sizes={`600px`} />
<Image src={image} sizes={`50vw`} />
<Image src={image} sizes={`12rem`} />
In the network tab you can see that the correct image sizes were fetched (Github example)
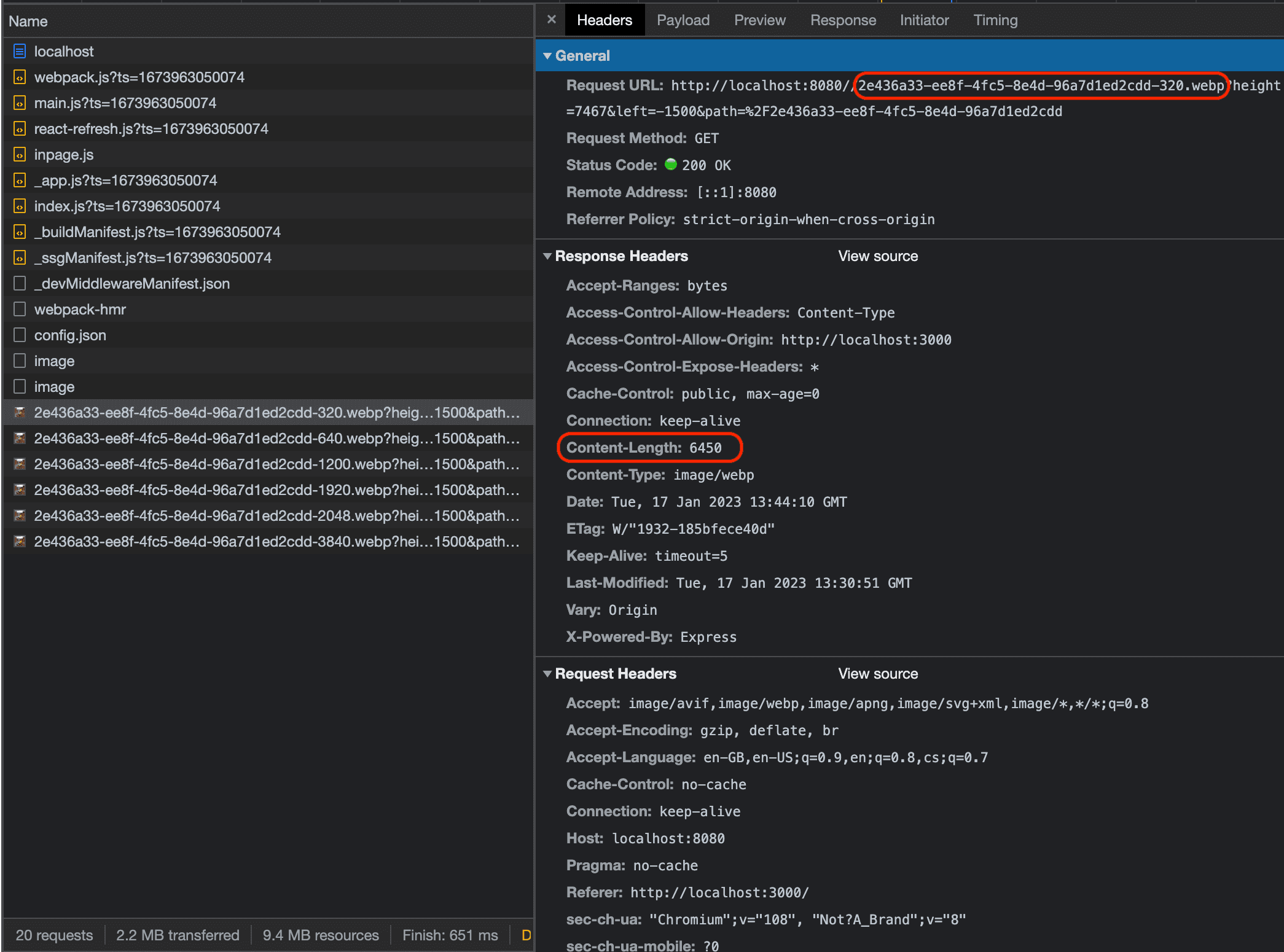
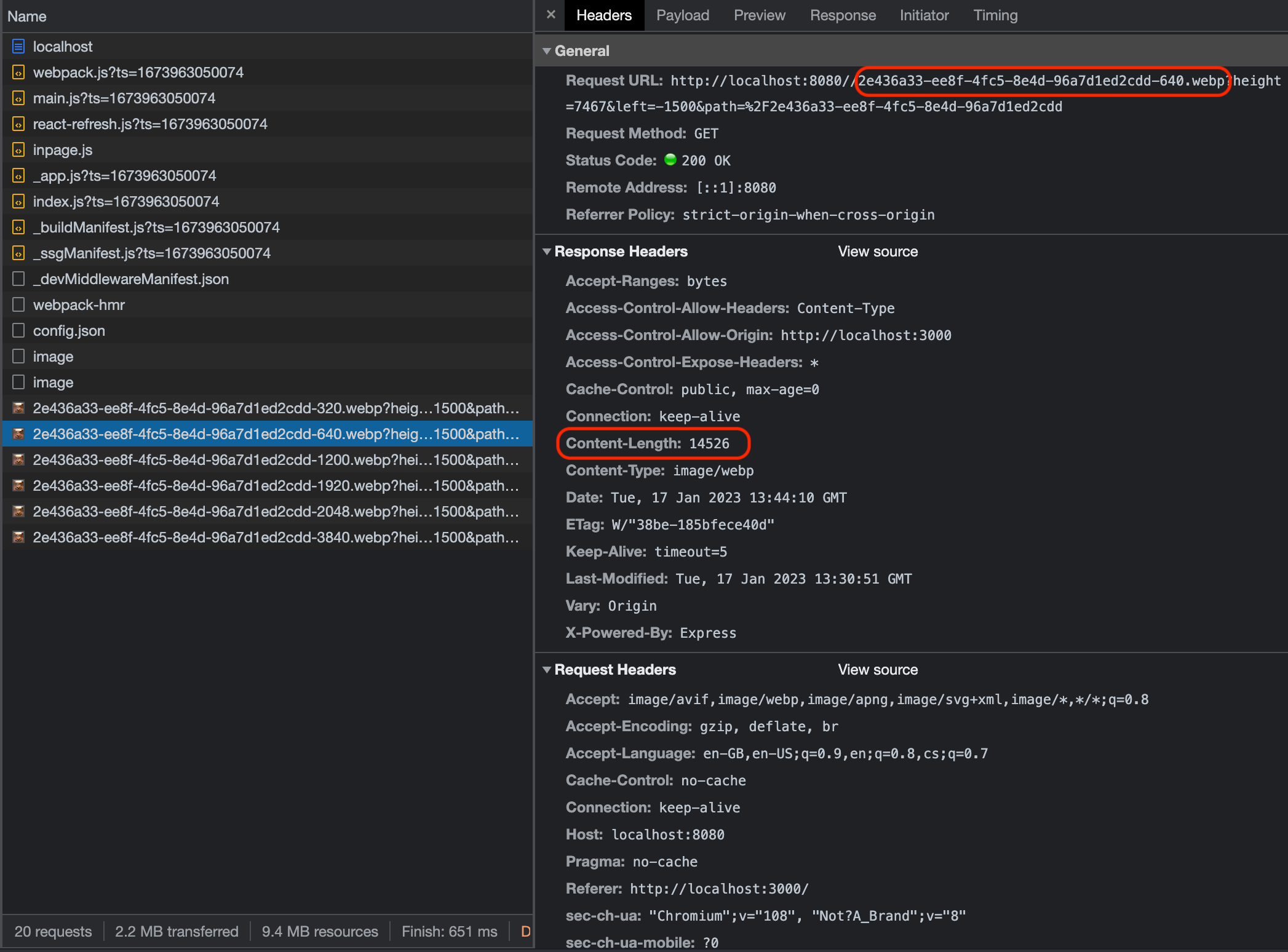
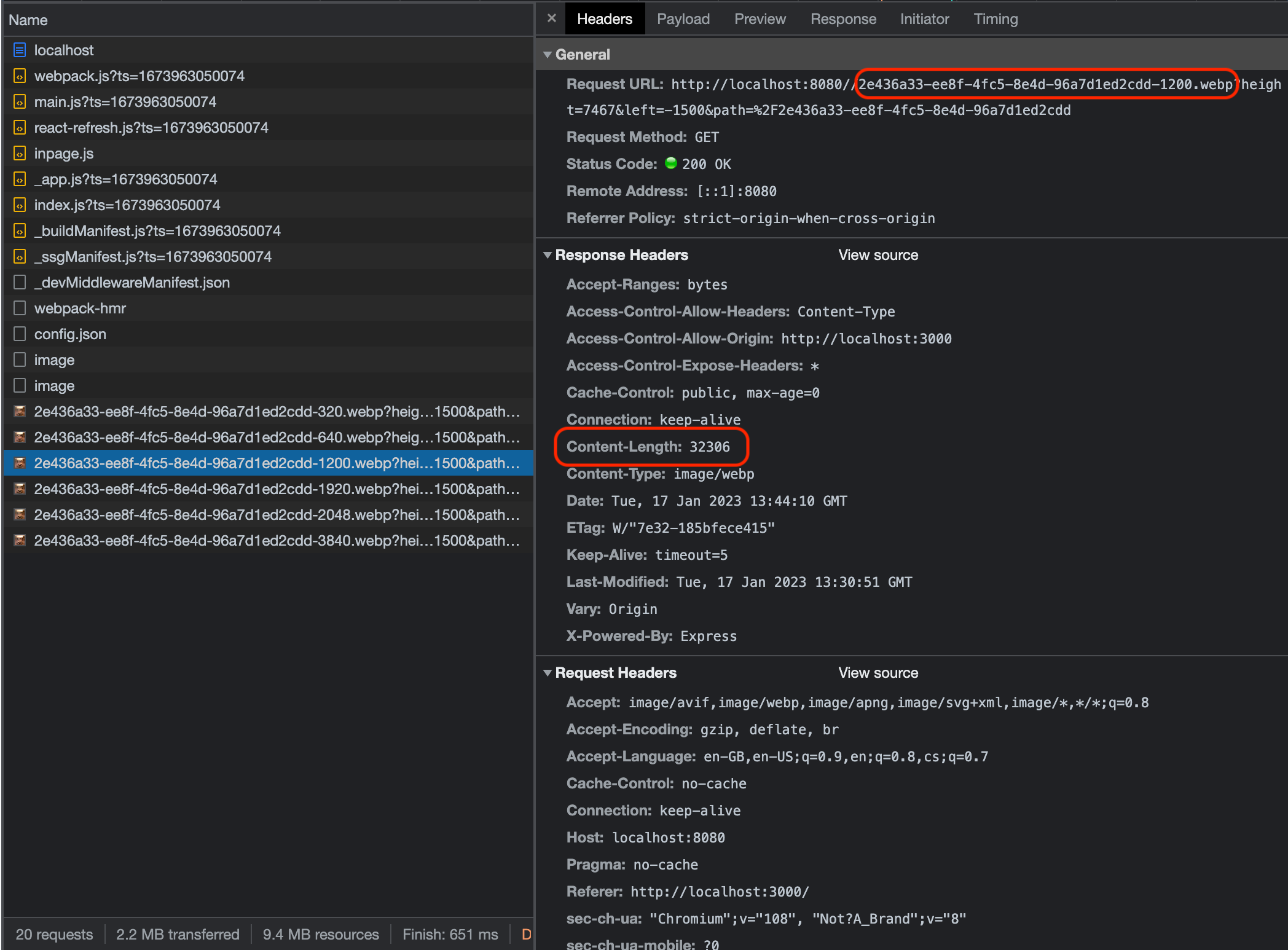
Edit Uploaded Image
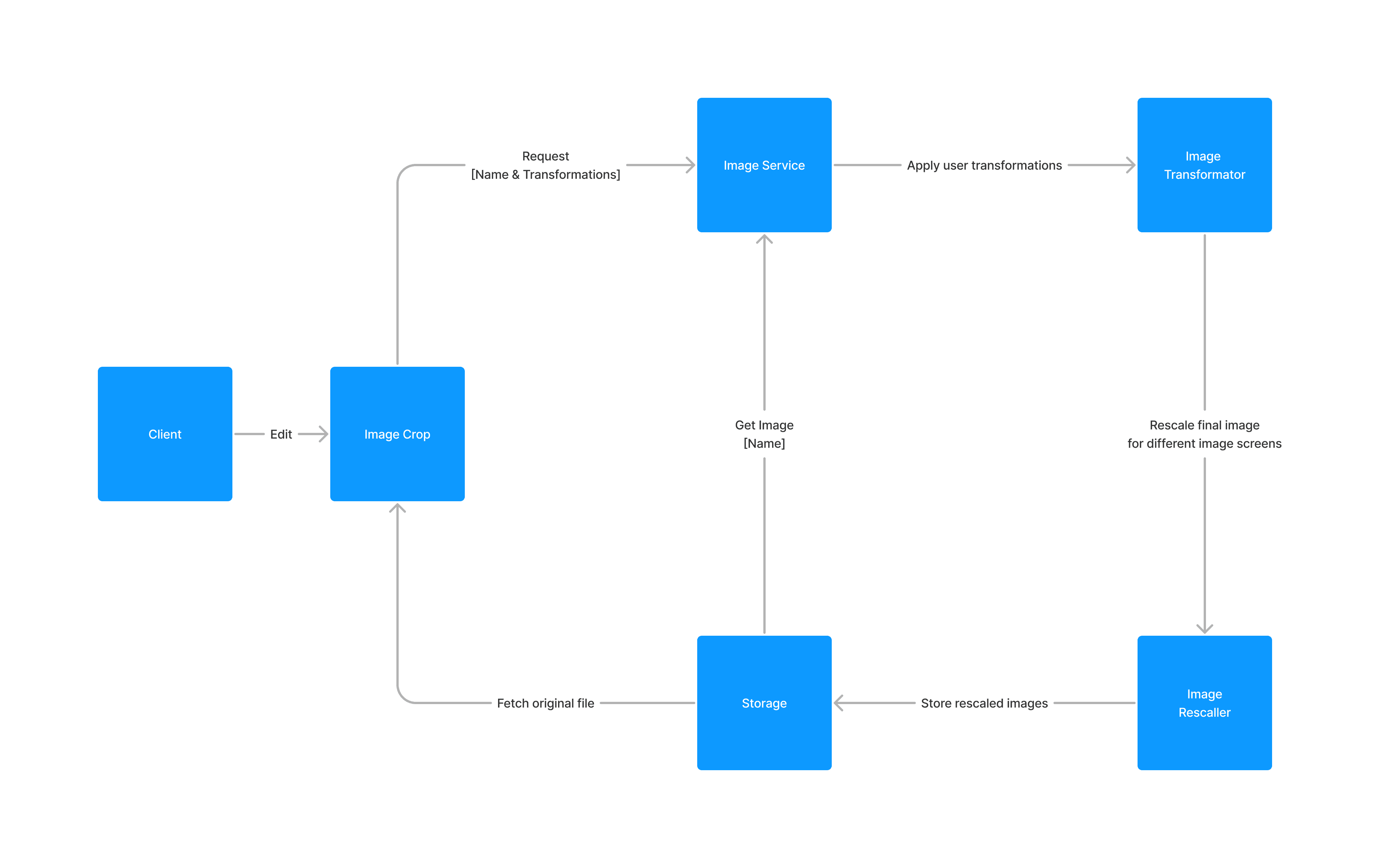
Editing an already uploaded image works similarly, but the image is not sent from the client as it is already in storage. The Image Crop component fetches the original image and based on file query parameters shows the correct state. The ImageService then based on the image name and new transformations updates images.
Kudos
Architecture, Code, and Configs come from Style Space
Connect with expert stylists, over 1-on-1 video styling sessions for clothing, hair, and makeup/skincare styling. Elevate your style, simplify getting ready and save time and money.